jquery 弹出层 弹窗
可以设置不同的背景和标题
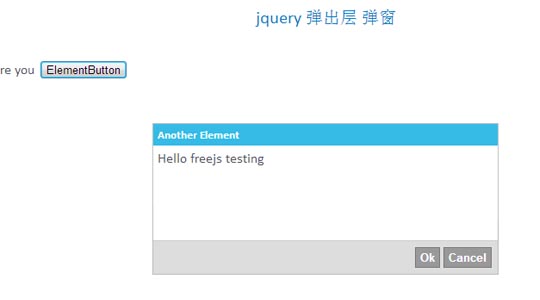
XML/HTML Code
- <button id="test">Alert</button>
- <div id="testElement"> Hello how are you
- <button>ElementButton</button>
- </div>
- <script src="includes/js/jquery.messagebox.js"></script>
- <script>
- $('#test').messagebox({
- title:'Title for freejs testing',
- messageText: 'Hello freejs testing',
- messageSubType: 'warning',
- messageType : 'alert',
- OkCallback: function() {
- alert('You pressed OK');
- },
- onClose: function() {
- alert('You closed the message box');
- },
- onCancel: function() {
- alert('You pressed cancel');
- }
- });
- $('#testElement').messagebox({
- title:'Another Element',
- messageText: 'Hello freejs testing',
- messageType : 'confirm',
- height: 100,
- width: 400,
- OkCallback: function() {
- alert('You pressed OK');
- },
- onClose: function() {
- alert('You closed the message box');
- },
- onCancel: function() {
- alert('You pressed cancel');
- }
- });
- </script>
JavaScript Code
- /*
- Custom Message box plugin for jQuery (https://github.com/mpsiva89/jquery-messagebox)
- Copyright (c) 2013 SivaKumar and Jeyadevan
- Licensed under the MIT license (http://opensource.org/licenses/mit-license.html)
- Version: 1.0.3
- */
- (function($, window, document, undefined) {
- var html = '<div class="msgboxOverlay"><div class="alert-box msgwrapper"><div class="information"><div class="msgcontainer"><div class="msgheader"><a href="javascript:void(0);" class="msgclose">Close</a><span>Message Box Title</span></div><div class="msg">Text</div><div class="msgfooter"></div></div></div></div></div>';
- var currentMessageBox;
- var buttonOk = '<button type="button" class="buttonOK">Ok</button>';
- var buttonCancel = '<button type="button" class="buttonCancel">Cancel</button>';
- var settings ;
- var pluginName = 'messagebox';
- function MessageBoxPlugin(element, options) {
- this.settings = settings;
- this.element = element;
- if (options) {
- this.options = options;
- }
- var current = this;
- $(this.element).click(function() {
- current.createMessageBox();
- $('body').prepend(currentMessageBox);
- current.intialize();
- });
- }
- MessageBoxPlugin.prototype.createMessageBox = function() {
- $.extend(this.settings, this.options);
- var temp = $(html);
- if(this.settings.messageElementId) {
- temp.find('.msg').html($(this.settings.messageElementId).html());
- } else {
- temp.find('.msg').html(this.settings.messageText);
- }
- if(this.settings.height)
- temp.find('.msg').height(this.settings.height);
- if(this.settings.width)
- temp.find('.msgcontainer').width(this.settings.width);
- temp.find('.msgheader span').html(this.settings.title);
- temp.find('.information').removeClass('information').addClass(this.settings.messageSubType);
- var buttons = buttonOk;
- if(this.settings.messageType === 'confirm') {
- buttons = buttons + buttonCancel;
- }
- temp.find('.msgfooter').html(buttons);
- currentMessageBox = $('<div>').append(temp.clone()).html();
- }
- MessageBoxPlugin.prototype.intialize = function() {
- var current = this;
- $.extend(current.settings, current.options);
- $('.msgboxOverlay .msgclose').off("click");
- $('.msgboxOverlay .msgclose').on("click", function() {
- $('.msgboxOverlay').remove();
- if(current.settings.onClose) {
- current.settings.onClose();
- }
- });
- $('.msgboxOverlay .buttonOK').off('click');
- $('.msgboxOverlay .buttonOK').on('click', function() {
- $('.msgboxOverlay').remove();
- if(current.settings.OkCallback) {
- current.settings.OkCallback();
- }
- });
- if(this.settings.messageType === 'confirm') {
- $('.msgboxOverlay .buttonCancel').off('click');
- $('.msgboxOverlay .buttonCancel').on('click', function() {
- $('.msgboxOverlay').remove();
- if(current.settings.onCancel) {
- current.settings.onCancel();
- }
- });
- }
- }
- $.fn.messagebox = function(options) {
- settings = {
- messageType: 'alert',
- messageSubType: 'information',
- title: 'Message',
- messageElementId: null,
- messageText: '',
- height: null,
- width: null,
- OkCallback : null,
- onClose: null,
- onCancel : null
- };
- return this.each(function () {
- if (!$.data(this, 'plugin_' + pluginName)) {
- $.data(this, 'plugin_' + pluginName,
- new MessageBoxPlugin( this, options ));
- }
- });
- };
- }(jQuery, window, document));
原文地址:http://www.freejs.net/article_jquerywenzi_148.html