jquery简单的无刷新提交和删除评论
数据库结构非常简单,就是一个id和content就可以了
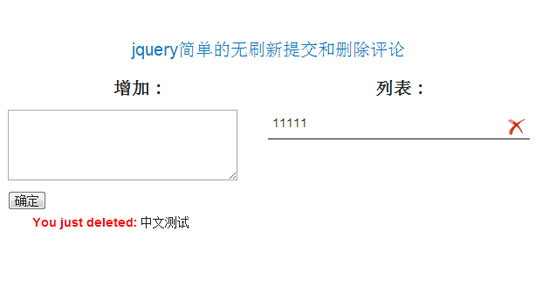
XML/HTML Code
- <script type="text/javascript">
- $(function(){
- //When the button with an id of submit is clicked (the submit button)
- $("#submit").click(function(){
- //Retrieve the contents of the textarea (the content)
- var formvalue = $("#content").val();
- //Build the URL that we will send
- var url = 'submit=1&content=' + formvalue;
- //Use jQuery's ajax function to send it
- $.ajax({
- type: "POST",
- url: "process.php",
- data: url,
- success: function(){
- //If successful , notify the user that it was added
- $("#msg").html("<p class='add'>You just added: <i>" + formvalue + "</i></p>");
- $("#content").val('');
- todolist();
- }
- });
- //We return false so when the button is clicked, it doesn't follow the action
- return false;
- });
- todolist();
- });
- function todolist(){
- $.ajax({
- url: "process.php",
- cache: false,
- success: function(html){
- $("#todolist").html(html);
- }
- });
- }
- </script>
- <style type="text/css">
- *{
- font: normal 14px/25px Helvetica, Arial, sans-serif;
- color: #212121;
- }
- h1{
- font-size: 20px;
- font-weight: bold;
- }
- h2{
- font-size: 16px;
- font-weight: bold;
- }
- .list td{
- border-bottom:1px solid;
- }
- .delete{
- position: relative;
- top: 5px;
- }
- .copy{
- padding: 100px;
- text-align: center;
- font-size: 11px;
- }
- .copy a{
- font-size: 11px;
- }
- .add{
- font-weight: bold;
- color: green;
- }
- .remove{
- font-weight: bold;
- color: red;
- }
- .msg{
- width:80%;
- }
- </style>
- <table border="0" width="600px" cellpadding="0" cellspacing="0" align="center">
- <tr valign="top">
- <td width="50%">
- <h2>增加 :</h2>
- <form id="form" action="process.php" method="post">
- <textarea name="content" id="content" cols="30" rows="3"></textarea> <br />
- <input type="submit" id="submit" name="submit" value="确定" />
- </form>
- <div class="msg" id="msg"></div>
- </td>
- <td width="50%">
- <h2>列表 :</h2>
- <div id="todolist"></div>
- </td>
- </tr>
- </table>
delete.php
PHP Code
- <?php
- //Connect to the database
- include 'conn.php';
- //delete.php?id=IdOfPost
- if($_POST['submit']){
- //if($_GET['id']){
- echo $id = $_POST['id'];
- //$id = $_GET['id'];
- //Delete the record of the post
- $delete = mysql_query("DELETE FROM `add_delete_record` WHERE `id` = '$id'");
- //Redirect the user
- header("Location:index.php");
- }
- ?>
process.php
PHP Code
- <?php
- //Connect to the database
- include 'conn.php';
- //Was the form submitted?
- if($_POST['submit']){
- //Map the content that was sent by the form a variable. Not necessary but it keeps things tidy.
- $content = $_POST['content'];
- //Insert the content into database
- $ins = mysql_query("INSERT INTO `add_delete_record` (content) VALUES ('$content')");
- //Redirect the user back to the index page
- header("Location:index.php");
- }
- /*Doesn't matter if the form has been posted or not, show the latest posts*/
- //Find all the notes in the database and order them in a descending order (latest post first).
- $find = mysql_query("SELECT * FROM `add_delete_record` ORDER BY id DESC");
- //Setup the un-ordered list
- echo '<table border="0" cellpadding="5" cellspacing="0" class="list" width="100%">';
- //Continue looping through all of them
- while($row = mysql_fetch_array($find)){
- //For each one, echo a list item giving a link to the delete page with it's id.
- echo '<tr><td valign="middle" width="90%">' . $row['content'] . ' </td>
- <td valign="middle" width="10%"><form id="form" action="delete.php?id=' . $row['id'] . '" method="post">
- <input class="todo_id" name="todo_id" type="hidden" value="' . $row['id'] . '" />
- <input class="todo_content" name="todo_content" type="hidden" value="' . $row['content'] . '" />
- <input type="image" src="images/delete.png" class="delete" name="delete" width="20px" />
- </form>
- </td></tr>';
- }
- //End the un-ordered list
- echo '</table>';
- ?>
- <script type="text/javascript">
- $(".delete").click(function(){
- //Retrieve the contents of the textarea (the content)
- var todo_id = $(this).parent().find(".todo_id").val();
- var todo_content = $(this).parent().find(".todo_content").val();
- //Build the URL that we will send
- var url = 'submit=1&id=' + todo_id;
- //Use jQuery's ajax function to send it
- $.ajax({
- type: "POST",
- url: "delete.php",
- data: url,
- success: function(){
- //If successful , notify the user that it was added
- $("#msg").html("<p class='remove'>You just deleted: <i>" + todo_content + "</i></p>");
- $("#content").val('');
- todolist();
- }
- });
- //We return false so when the button is clicked, it doesn't follow the action
- return false;
- });
- </script>
原文地址:http://www.freejs.net/article_biaodan_102.html