jquery php多文件上传
多文件上传
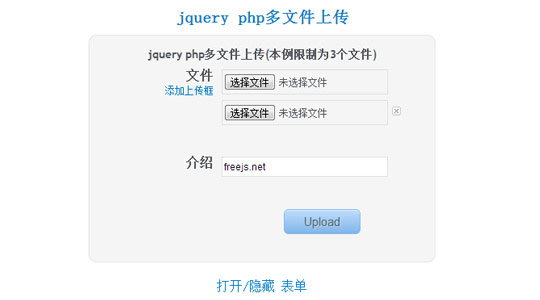
index.php
PHP Code
- <div id="uploaderform">
- <form action="upload.php" method="post" enctype="multipart/form-data" name="UploadForm" id="UploadForm">
- <h1>jquery php多文件上传(本例限制为3个文件)</h1>
- <label>文件
- <span class="small"><a href="#" id="AddMoreFileBox">添加上传框</a></span>
- </label>
- <div id="AddFileInputBox"><input id="fileInputBox" style="margin-bottom: 5px;" type="file" name="file[]"/></div>
- <div class="sep_s"></div>
- <label>介绍
- </label>
- <div><input name="username" type="text" id="username" value="freejs.net" /></div>
- <button type="submit" class="button" id="SubmitButton">Upload</button>
- <div id="progressbox"><div id="progressbar"></div ><div id="statustxt">0%</div ></div>
- </form>
- </div>
- <div id="uploadResults">
- <div align="center" style="margin:20px;"><a href="#" id="ShowForm">打开/隐藏 表单</a></div>
- <div id="output"></div>
- </div>
js文件
JavaScript Code
- <script type="text/javascript">
- $(document).ready(function() {
- //elements
- var progressbox = $('#progressbox'); //progress bar wrapper
- var progressbar = $('#progressbar'); //progress bar element
- var statustxt = $('#statustxt'); //status text element
- var submitbutton = $("#SubmitButton"); //submit button
- var myform = $("#UploadForm"); //upload form
- var output = $("#output"); //ajax result output element
- var completed = '0%'; //initial progressbar value
- var FileInputsHolder = $('#AddFileInputBox'); //Element where additional file inputs are appended
- var MaxFileInputs = 3; //Maximum number of file input boxs
- // adding and removing file input box
- var i = $("#AddFileInputBox div").size() + 1;
- $("#AddMoreFileBox").click(function () {
- event.returnValue = false;
- if(i < MaxFileInputs)
- {
- $('<span><input type="file" id="fileInputBox" size="20" name="file[]" class="addedInput" value=""/><a href="#" class="removeclass small2"><img src="images/close_icon.gif" border="0" /></a></span>').appendTo(FileInputsHolder);
- i++;
- }
- return false;
- });
- $("body").on("click",".removeclass", function(e){
- event.returnValue = false;
- if( i > 1 ) {
- $(this).parents('span').remove();i--;
- }
- });
- $("#ShowForm").click(function () {
- $("#uploaderform").slideToggle(); //Slide Toggle upload form on click
- });
- $(myform).ajaxForm({
- beforeSend: function() { //brfore sending form
- submitbutton.attr('disabled', ''); // disable upload button
- statustxt.empty();
- progressbox.show(); //show progressbar
- progressbar.width(completed); //initial value 0% of progressbar
- statustxt.html(completed); //set status text
- statustxt.css('color','#000'); //initial color of status text
- },
- uploadProgress: function(event, position, total, percentComplete) { //on progress
- progressbar.width(percentComplete + '%') //update progressbar percent complete
- statustxt.html(percentComplete + '%'); //update status text
- if(percentComplete>50)
- {
- statustxt.css('color','#fff'); //change status text to white after 50%
- }else{
- statustxt.css('color','#000');
- }
- },
- complete: function(response) { // on complete
- output.html(response.responseText); //update element with received data
- myform.resetForm(); // reset form
- submitbutton.removeAttr('disabled'); //enable submit button
- progressbox.hide(); // hide progressbar
- $("#uploaderform").slideUp(); // hide form after upload
- }
- });
- });
- </script>
uoload.php
PHP Code
- <noscript>
- <div align="center"><a href="index.php">Go Back To Upload Form</a></div><!-- If javascript is disabled -->
- </noscript>
- <?php
- //If you face any errors, increase values of "post_max_size", "upload_max_filesize" and "memory_limit" as required in php.ini
- //Some Settings
- $ThumbSquareSize = 200; //Thumbnail will be 200x200
- $BigImageMaxSize = 500; //Image Maximum height or width
- $ThumbPrefix = "thumb_"; //Normal thumb Prefix
- $DestinationDirectory = '../upload/'; //Upload Directory ends with / (slash)
- $Quality = 90;
- //ini_set('memory_limit', '-1'); // maximum memory!
- foreach($_FILES as $file)
- {
- // some information about image we need later.
- $ImageName = $file['name'];
- $ImageSize = $file['size'];
- $TempSrc = $file['tmp_name'];
- $ImageType = $file['type'];
- if (is_array($ImageName))
- {
- $c = count($ImageName);
- echo '<ul>';
- for ($i=0; $i < $c; $i++)
- {
- $processImage = true;
- $RandomNumber = rand(0, 9999999999); // We need same random name for both files.
- if(!isset($ImageName[$i]) || !is_uploaded_file($TempSrc[$i]))
- {
- echo '<div class="error">Error occurred while trying to process <strong>'.$ImageName[$i].'</strong>, may be file too big!</div>'; //output error
- }
- else
- {
- //Validate file + create image from uploaded file.
- switch(strtolower($ImageType[$i]))
- {
- case 'image/png':
- $CreatedImage = imagecreatefrompng($TempSrc[$i]);
- break;
- case 'image/gif':
- $CreatedImage = imagecreatefromgif($TempSrc[$i]);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- $CreatedImage = imagecreatefromjpeg($TempSrc[$i]);
- break;
- default:
- $processImage = false; //image format is not supported!
- }
- //get Image Size
- list($CurWidth,$CurHeight)=getimagesize($TempSrc[$i]);
- //Get file extension from Image name, this will be re-added after random name
- $ImageExt = substr($ImageName[$i], strrpos($ImageName[$i], '.'));
- $ImageExt = str_replace('.','',$ImageExt);
- //Construct a new image name (with random number added) for our new image.
- $NewImageName = $RandomNumber.'.'.$ImageExt;
- //Set the Destination Image path with Random Name
- $thumb_DestRandImageName = $DestinationDirectory.$ThumbPrefix.$NewImageName; //Thumb name
- $DestRandImageName = $DestinationDirectory.$NewImageName; //Name for Big Image
- //Resize image to our Specified Size by calling resizeImage function.
- if($processImage && resizeImage($CurWidth,$CurHeight,$BigImageMaxSize,$DestRandImageName,$CreatedImage,$Quality,$ImageType[$i]))
- {
- //Create a square Thumbnail right after, this time we are using cropImage() function
- if(!cropImage($CurWidth,$CurHeight,$ThumbSquareSize,$thumb_DestRandImageName,$CreatedImage,$Quality,$ImageType[$i]))
- {
- echo 'Error Creating thumbnail';
- }
- /*
- At this point we have succesfully resized and created thumbnail image
- We can render image to user's browser or store information in the database
- For demo, we are going to output results on browser.
- */
- //Get New Image Size
- list($ResizedWidth,$ResizedHeight)=getimagesize($DestRandImageName);
- echo '<table width="100%" border="0" cellpadding="4" cellspacing="0">';
- echo '<tr>';
- echo '<td align="center"><img src="../upload/'.$ThumbPrefix.$NewImageName.'" alt="Thumbnail" height="'.$ThumbSquareSize.'" width="'.$ThumbSquareSize.'"></td>';
- echo '</tr><tr>';
- echo '<td align="center"><img src="../upload/'.$NewImageName.'" alt="Resized Image" height="'.$ResizedHeight.'" width="'.$ResizedWidth.'"></td>';
- echo '</tr>';
- echo '</table>';
- /*
- // Insert info into database table!
- mysql_query("INSERT INTO myImageTable (ImageName, ThumbName, ImgPath)
- VALUES ($DestRandImageName, $thumb_DestRandImageName, '../upload/')");
- */
- }else{
- echo '<div class="error">Error occurred while trying to process <strong>'.$ImageName[$i].'</strong>! Please check if file is supported</div>'; //output error
- }
- }
- }
- echo '</ul>';
- }
- }
- // This function will proportionally resize image
- function resizeImage($CurWidth,$CurHeight,$MaxSize,$DestFolder,$SrcImage,$Quality,$ImageType)
- {
- //Check Image size is not 0
- if($CurWidth <= 0 || $CurHeight <= 0)
- {
- return false;
- }
- //Construct a proportional size of new image
- $ImageScale = min($MaxSize/$CurWidth, $MaxSize/$CurHeight);
- $NewWidth = ceil($ImageScale*$CurWidth);
- $NewHeight = ceil($ImageScale*$CurHeight);
- if($CurWidth < $NewWidth || $CurHeight < $NewHeight)
- {
- $NewWidth = $CurWidth;
- $NewHeight = $CurHeight;
- }
- $NewCanves = imagecreatetruecolor($NewWidth, $NewHeight);
- // Resize Image
- if(imagecopyresampled($NewCanves, $SrcImage,0, 0, 0, 0, $NewWidth, $NewHeight, $CurWidth, $CurHeight))
- {
- switch(strtolower($ImageType))
- {
- case 'image/png':
- imagepng($NewCanves,$DestFolder);
- break;
- case 'image/gif':
- imagegif($NewCanves,$DestFolder);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- imagejpeg($NewCanves,$DestFolder,$Quality);
- break;
- default:
- return false;
- }
- if(is_resource($NewCanves)) {
- imagedestroy($NewCanves);
- }
- return true;
- }
- }
- //This function corps image to create exact square images, no matter what its original size!
- function cropImage($CurWidth,$CurHeight,$iSize,$DestFolder,$SrcImage,$Quality,$ImageType)
- {
- //Check Image size is not 0
- if($CurWidth <= 0 || $CurHeight <= 0)
- {
- return false;
- }
- //abeautifulsite.net has excellent article about "Cropping an Image to Make Square"
- //http://www.abeautifulsite.net/blog/2009/08/cropping-an-image-to-make-square-thumbnails-in-php/
- if($CurWidth>$CurHeight)
- {
- $y_offset = 0;
- $x_offset = ($CurWidth - $CurHeight) / 2;
- $square_size = $CurWidth - ($x_offset * 2);
- }else{
- $x_offset = 0;
- $y_offset = ($CurHeight - $CurWidth) / 2;
- $square_size = $CurHeight - ($y_offset * 2);
- }
- $NewCanves = imagecreatetruecolor($iSize, $iSize);
- if(imagecopyresampled($NewCanves, $SrcImage,0, 0, $x_offset, $y_offset, $iSize, $iSize, $square_size, $square_size))
- {
- switch(strtolower($ImageType))
- {
- case 'image/png':
- imagepng($NewCanves,$DestFolder);
- break;
- case 'image/gif':
- imagegif($NewCanves,$DestFolder);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- imagejpeg($NewCanves,$DestFolder,$Quality);
- break;
- default:
- return false;
- }
- if(is_resource($NewCanves)) {
- imagedestroy($NewCanves);
- }
- return true;
- }
- }
原文地址:http://www.freejs.net/article_biaodan_45.html