带图片的select多选框美化
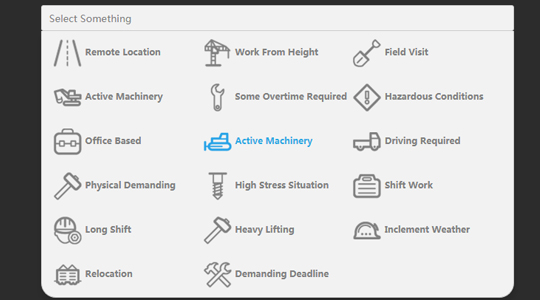
XML/HTML Code
- <select name="work-condition" id="work-condition" class="form-control">
- <option value="1" icon="iw-way">Remote Location</option>
- <option value="2" icon="iw-crane">Work From Height</option>
- <option value="3" icon="iw-scope">Field Visit</option>
- <option value="4" icon="iw-excavator">Active Machinery</option>
- <option value="5" icon="iw-key">Some Overtime Required</option>
- <option value="6" icon="iw-warning">Hazardous Conditions</option>
- <option value="7" icon="iw-bag">Office Based</option>
- <option value="8" icon="iw-dozer">Active Machinery</option>
- <option value="9" icon="iw-pickup">Driving Required</option>
- <option value="10" icon="iw-hammer">Physical Demanding</option>
- <option value="11" icon="iw-drill">High Stress Situation</option>
- <option value="12" icon="iw-note">Shift Work</option>
- <option value="13" icon="iw-head">Long Shift</option>
- <option value="14" icon="iw-hammer-o">Heavy Lifting</option>
- <option value="15" icon="iw-hat">Inclement Weather</option>
- <option value="16" icon="iw-building">Relocation</option>
- <option value="17" icon="iw-tools">Demanding Deadline</option>
- </select>
JavaScript Code
- class SelectBeauty {
- constructor(data)
- {
- this.el = data.el;
- this.placeholder = (typeof data.placeholder !== "undefined") ? data.placeholder : "Select Something";
- this.length = (typeof data.length !== "undefined") ? data.length : 3;
- this.max = (typeof data.max !== "undefined") ? data.max : false;
- this.selected = {};
- this.tempData = {};
- this.getTemporaryData();
- this.getHtml();
- this.hideSelect();
- this.renderView();
- this.buttonListener();
- this.toggleListener();
- this.selectListener();
- }
- // get data from select
- getTemporaryData()
- {
- let _this = this;
- $.each($(this.el).children(), function(i, r) {
- let el = $(r);
- let data = {
- text: el.text(),
- icon: el.attr('icon')
- }
- _this.tempData[el.attr('value')] = data;
- });
- }
- // hide select with add attribute multiple
- hideSelect()
- {
- $(this.el).hide();
- $(this.el).attr('multiple', true);
- }
- renderView()
- {
- $(this.el).parent().append(this.htmlBeauty);
- }
- htmlData()
- {
- let html = '';
- for(name in this.tempData) {
- html += `
- <li>
- <a href="#" data-value="${name}"><i class="iw ${this.tempData[name].icon}"></i> ${this.tempData[name].text}</a>
- </li>
- `;
- }
- return html;
- }
- getHtml()
- {
- this.htmlBeauty = `<div class="select-beauty" data-instance="${this.el}">
- <button class="button-sb button-sb-block">${this.placeholder}</button>
- <ul class="hide">
- ${this.htmlData()}
- </ul>
- </div>`;
- }
- toggleListener()
- {
- let _this = this;
- $(document).click(function(ev) {
- let el = $('[data-instance="'+_this.el+'"] ul');
- el.addClass('hide');
- });
- $('[data-instance="'+this.el+'"] ul').click(function(ev) {
- ev.stopPropagation();
- });
- }
- buttonListener()
- {
- $(document).find('[data-instance="'+this.el+'"]').on('click', 'button', function(ev) {
- ev.preventDefault();
- ev.stopPropagation();
- $(this).next('ul').toggleClass('hide');
- });
- }
- selectListener()
- {
- let _this = this;
- $(document).find('[data-instance="'+this.el+'"] ul').on('click', 'a', function(ev) {
- ev.stopPropagation();
- ev.preventDefault();
- let el = $(this);
- let id = el.attr('data-value');
- let parent = el.parent();
- if(parent.hasClass('active')) {
- delete _this.selected[id];
- parent.removeClass('active');
- } else {
- if(_this.max && _this.countObject(_this.selected) >= _this.max) return false;
- _this.selected[id] = _this.tempData[id];
- parent.addClass('active');
- }
- _this.filter();
- });
- }
- countObject(obj)
- {
- return Object.keys(obj).length;
- }
- filter()
- {
- let arr = [];
- let btnText = [];
- let btnElement = $('[data-instance="'+this.el+'"] button');
- for(name in this.selected) {
- arr.push(name);
- btnText.push(this.selected[name].text);
- }
- $(this.el).val(arr);
- if(btnText.length < 1) {
- btnElement.text(this.placeholder);
- } else if(btnText.length > this.length) {
- btnElement.text("("+btnText.length+") Selected");
- } else {
- btnElement.text(btnText.join(', '));
- }
- }
- reload()
- {
- this.selected = {};
- this.tempData = {};
- $(this.el).val([]);
- this.getTemporaryData();
- $(document).find('[data-instance="'+this.el+'"] ul').html('');
- $(document).find('[data-instance="'+this.el+'"] ul').html(this.htmlData());
- }
- }
原文地址:http://www.freejs.net/article_biaodan_783.html