各种不同演示的图片滚动
包括是否可以循环,设置默认显示位置等不同情形
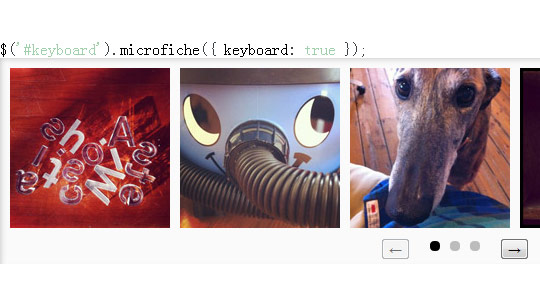
JavaScript Code
- <script>
- function example(id) {
- document.write(
- '<div class="example" id="' + id + '">' +
- '<div>' +
- '<ul>' +
- '<li><img src="images/1.jpg" width="160" height="160"></li>' +
- '<li><img src="images/2.jpg" width="160" height="160"></li>' +
- '<li><img src="images/3.jpg" width="160" height="160"></li>' +
- '<li><img src="images/4.jpg" width="160" height="160"></li>' +
- '<li><img src="images/5.jpg" width="160" height="160"></li>' +
- '<li><img src="images/6.jpg" width="160" height="160"></li>' +
- '<li><img src="images/7.jpg" width="160" height="160"></li>' +
- '<li><img src="images/8.jpg" width="160" height="160"></li>' +
- '<li><img src="images/9.jpg" width="160" height="160"></li>' +
- '<li><img src="images/10.jpg" width="160" height="160"></li>' +
- '<li><img src="images/11.jpg" width="160" height="160"></li>' +
- '<li><img src="images/12.jpg" width="160" height="160"></li>' +
- '<li><img src="images/13.jpg" width="160" height="160"></li>' +
- '<li><img src="images/14.jpg" width="160" height="160"></li>' +
- '</ul>' +
- '</div>' +
- '</div>'
- );
- }
- </script>
XML/HTML Code
- <h3>Defaults</h3>
- <div class="demo">
- <p><code class="prettyprint">$('#default').microfiche();</code></p>
- <script>example('default')</script>
- <script>$('#default').microfiche()</script>
- </div>
- <h3>Options</h3>
- <div class="demo">
- <p><code class="prettyprint">$('#cyclic').microfiche({ cyclic: true });</code></p>
- <script>example('cyclic')</script>
- <script>$('#cyclic').microfiche({ cyclic: true })</script>
- </div>
- <div class="demo">
- <p><code class="prettyprint">$('#buttons').microfiche({ buttons: false });</code></p>
- <script>example('buttons')</script>
- <script>$('#buttons').microfiche({ buttons: false })</script>
- </div>
- <div class="demo">
- <p><code class="prettyprint">$('#bullets').microfiche({ bullets: false });</code></p>
- <script>example('bullets')</script>
- <script>$('#bullets').microfiche({ bullets: false })</script>
- </div>
- <div class="demo">
- <p><code class="prettyprint">$('#keyboard').microfiche({ keyboard: true });</code></p>
- <script>example('keyboard')</script>
- <script>$('#keyboard').microfiche({ keyboard: true })</script>
- </div>
- <div class="demo">
- <p><code class="prettyprint">$('#click').microfiche({ clickToAdvance: true });</code></p>
- <script>example('click')</script>
- <script>$('#click').microfiche({ clickToAdvance: true })</script>
- </div>
- <h3>Commands</h3>
- <p>Commands are passed to the microfiche method as options, and may be passed at any point.<br>
- In this example, the <code class="prettyprint">slideByPages(1)</code> command is performed immediately
- after Microfiche has finished setting up.</p>
- <div class="demo">
- <p><code class="prettyprint">$('#commands').microfiche({ slideByPages: 1 });</code></p>
- <script>example('commands')</script>
- <script>$('#commands').microfiche({ slideByPages: 1 })</script>
- <p>
- <button onClick="$('#commands').microfiche({ slideByPages: -1 }); return false;">Run →</button> <code class="prettyprint">$('#commands').microfiche({ slideByPages: -1 })</code><br>
- <button onClick="$('#commands').microfiche({ slideByPages: 1 }); return false;">Run →</button> <code class="prettyprint">$('#commands').microfiche({ slideByPages: 1 })</code><br>
- <button onClick="$('#commands').microfiche({ slideToPoint: 0 }); return false;">Run →</button> <code class="prettyprint">$('#commands').microfiche({ slideToPoint: 0 })</code><br>
- <button onClick="$('#commands').microfiche({ jumpToPoint: 0 }); return false;">Run →</button> <code class="prettyprint">$('#commands').microfiche({ jumpToPoint: 0 })</code><br>
- <button onClick="$('#commands').microfiche({ jumpToPage: 2 }); return false;">Run →</button> <code class="prettyprint">$('#commands').microfiche({ jumpToPage: 2 })</code>
- </p>
- </div>
- <h3>Events</h3>
- <p>Microfiche emits the following events:</p>
- <ul>
- <li><code class="prettyprint">'microfiche:willMove'</code></li>
- <li><code class="prettyprint">'microfiche:didMove'</code></li>
- </ul>
- <p>You can listen for them in the usual way:</p>
- <div class="demo">
- <p><pre class="prettyprint">
- $('#events').microfiche().on('microfiche:willMove microfiche:didMove', function(event) {
- $('#events-console').html(event.type);
- });
- </pre></p>
- <script>example('events')</script>
- <script>
- $('#events').microfiche().on('microfiche:willMove microfiche:didMove', function(event) {
- $('#events-console').html(event.type);
- });
- </script>
- <p><strong>Event received:</strong> <code id="events-console">-</code></p>
- </div>
- <h3>Talking to Microfiche Directly</h3>
- <p>Microfiche has some useful methods that return values, and so cannot
- be used through the aforementioned jQuery style syntax. The microfiche
- object itself is available via jQuery’ <code>data</code> method.</p>
- <p><code class="prettyprint">$('.my-element').data('microfiche');</code></p>
- <h3>Methods</h3>
- <div class="demo">
- <p><pre class="prettyprint">
- var m = $('#methods').microfiche().data('microfiche');
- m.currentPageIndex() // returns 0-index of the current page
- m.totalPageCount() // returns the number of pages as an integer
- m.min() // returns the minimum (left-most) position
- m.max() // returns the maximum (right-most) position
- </pre></p>
- <script>example('methods')</script>
- <script>
- var m = $('#methods').microfiche().data('microfiche');
- document.write('<pre class="prettyprint">' +
- 'currentPageIndex: ' + m.currentPageIndex() + '<br>' +
- ' totalPageCount: ' + m.totalPageCount() + '<br>' +
- ' min: ' + m.min() + '<br>' +
- ' max: ' + m.max() +
- '</pre>');
- </script>
- </div>
- <h3>Autoplay</h3>
- <p>To have Microfiche pause rotation when the user hovers over the
- carousel, set <code>autopause</code> to <code>true</code>.</p>
- <div class="demo">
- <p><pre class="prettyprint">
- $('#autoplay').microfiche({
- cyclic: true,
- autoplay: 3,
- autopause: true
- });
- </pre></p>
- <script>example('autoplay')</script>
- <script>
- $('#autoplay').microfiche({ cyclic: true, autoplay: 3, autopause: true });
- </script>
- </div>
- <script>prettyPrint()</script>
原文地址:http://www.freejs.net/article_jquerytupiantexiao_175.html
最近更新
- jQuery json 无刷新翻页 ...
- 纯css3带倒影效果的图片翻转特效
- jQuery网站右下角悬浮留言表单代...
- jquery宽屏焦点图片动画轮播代码
- jquery带缩略图可触摸焦点图
- jquery无刷新分页
我爱薅羊毛
点击最多
广告赞助
相关文章
- 超简单的jquery 左右滚动图片,箭头控制左右滚...
- jQuery 几种不同的slide滑动banner...
- jQuery响应式图片跑马灯 无缝滚动
- jQuery支持鼠标滚轮缩放以及点击按钮缩放图片插...
- scrollable各种无缝滚动演示,包括水平/垂...
- 支持PC和移动端的滑块式验证码 拖动拼图式验证码